JaveScript 数据类型
JavaScript 的基本数据类型
最新的 ECMAScript 定义了6种原始数据类型以及 Object
对象:
原始数据类型:
string
(字符串)
number
(数字、NaN)
boolean
(布尔类型,只有两个值:true、false)
null
(表示为空的特殊关键字)
undefined
(表示定义的变量未被赋值)
symbol
(ES6新增,表示独一无二的值)
引用数据类型:
原始值和引用值的区别
原始值:
- 原始值是存储在栈(stack)中的简单数据段,它们的值直接存储在变量访问的位置;
- 所有的原始数据都是不可改变的。
引用值:
- 引用值是存储在堆(heap)中的对象,存储在变量处的值是一个指针(point),指向存储变量的内存处;
- JavaScript的引用值即对象。
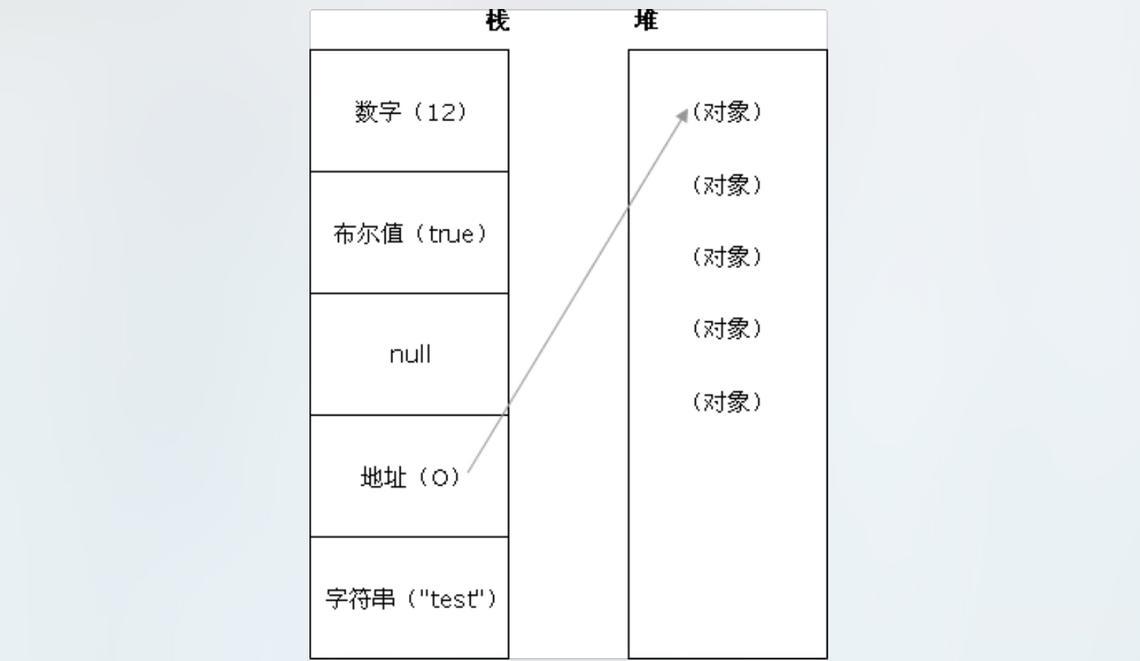
typeof 操作符
typeof
操作符返回一个字符串,判断给定表达式的数据类型。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34
| > typeof undefined 'undefined' > typeof null 'object' > typeof true 'boolean' > typeof 12 'number' > typeof Number(1) // 不推荐使用 'number' > typeof Infinity 'number' > typeof NaN 'number' > typeof 1/0 NaN > typeof 'hello world' 'string' > typeof String('abc') 'string' > typeof Symbol() 'symbol' > typeof {a:1} 'object' > typeof [1,2,3] 'object' > typeof function(){} 'function' > typeof new Boolean(true) // 不推荐使用 'object' > typeof new Number(1) // 不推荐使用 'object' > typeof new String('123') // 不推荐使用 'object'
|
数据类型的转换
JavaScript是一种动态类型语言(dynamically typed language)。这意味着你声明变量时可以不必指定数据类型,而数据类型会在脚本执行时根据需要自动转换。
强制转换
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| Number("123") Number("") Number("0x11") Number("0b11") Number("0o11") Number("foo") Number("100a") Number(null) Number(undefined) Number(new Date("2018-01-01")) Number(true) Number(false) Number([1,2,3]) Number([5]) Number({a:1}) Number(" 12.04 ")
|
1 2 3 4 5 6 7 8 9
| String(123) String(true) String(false) String([1,2,3]) String({a:1}) String(null) String(undefined) String(new Date("2018-01-01")) String(NaN)
|
1 2 3 4 5 6 7 8
| Boolean(undefined) Boolean(null) Boolean(0) Boolean(NaN) Boolean('') Boolean({}) Boolean([]) Boolean(new Boolean(false))
|
自动转换
1 2 3 4 5 6 7 8 9 10 11
| 123 + 'abc'
if ('abc') { console.log('hello') }
+ {foo: 'bar'} - [1, 2, 3]
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| if ( !undefined && !null && !0 && !NaN && !'' ) { console.log('true'); }
expression ? true : false
!! expression
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| '5' + 1 '5' + true '5' + false '5' + {} '5' + [] '5' + function (){} '5' + undefined '5' + null
var obj = { width: '100' };
obj.width + 20
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| '5' - '2' '5' * '2' true - 1 false - 1 '1' - 1 '5' * [] false / '5' 'abc' - 1 null + 1 undefined + 1
+'abc' -'abc' +true -false
|
参考资料
语法和数据类型 [https://developer.mozilla.org/zh-CN/docs/Web/JavaScript/Guide/Grammar_and_Types]
typeof [https://developer.mozilla.org/zh-CN/docs/Web/JavaScript/Reference/Operators/typeof]
ECMAScript 原始值和引用值 [http://www.w3school.com.cn/js/pro_js_value.asp]
数据类型转换 – JavaScript 标准参考教程(alpha)[http://javascript.ruanyifeng.com/grammar/conversion.html]